Shot-Bot 2.0
- Josh Leipheimer
- Dec 19, 2015
- 8 min read
Introducing the Shot-Bot 2.0, a completely autonomous bartender that mixes cocktails ordered from your smartphone completely autonomously. This robotic bartender carries your glass to certain pouring stations to make you the perfect cocktail of your drunken desires. This project is powered using a Nema 17 stepper motor, DC motor actuators, and an Arduino as the main controller. Cocktails are ordered via a bluetooth app that can be downloaded from a samsung smartphone.
This project was coded using C based language as part of the Arduino IDE software. Arduino is a microprocessor that is great at controlling electronic projects such as this because of it's input and output pins. The downside of Arduino is that it can not handle multiple tasks at once. For this reason I had to use two Arduinos, one for the motor control and one for the light control.
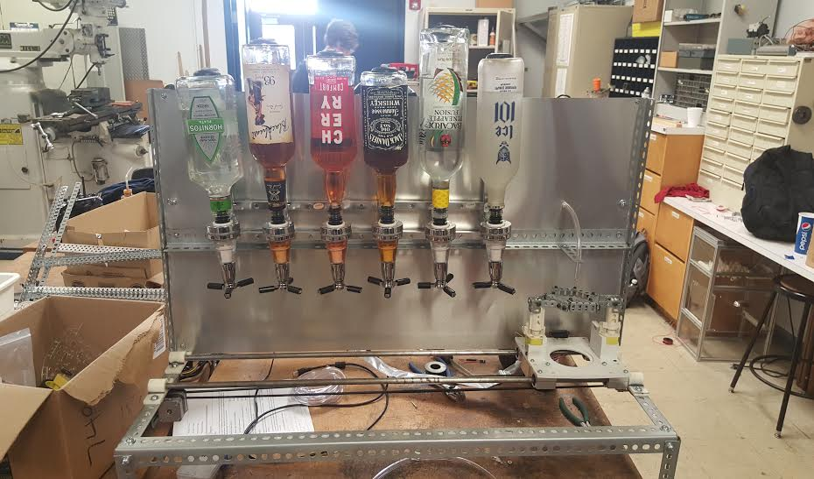
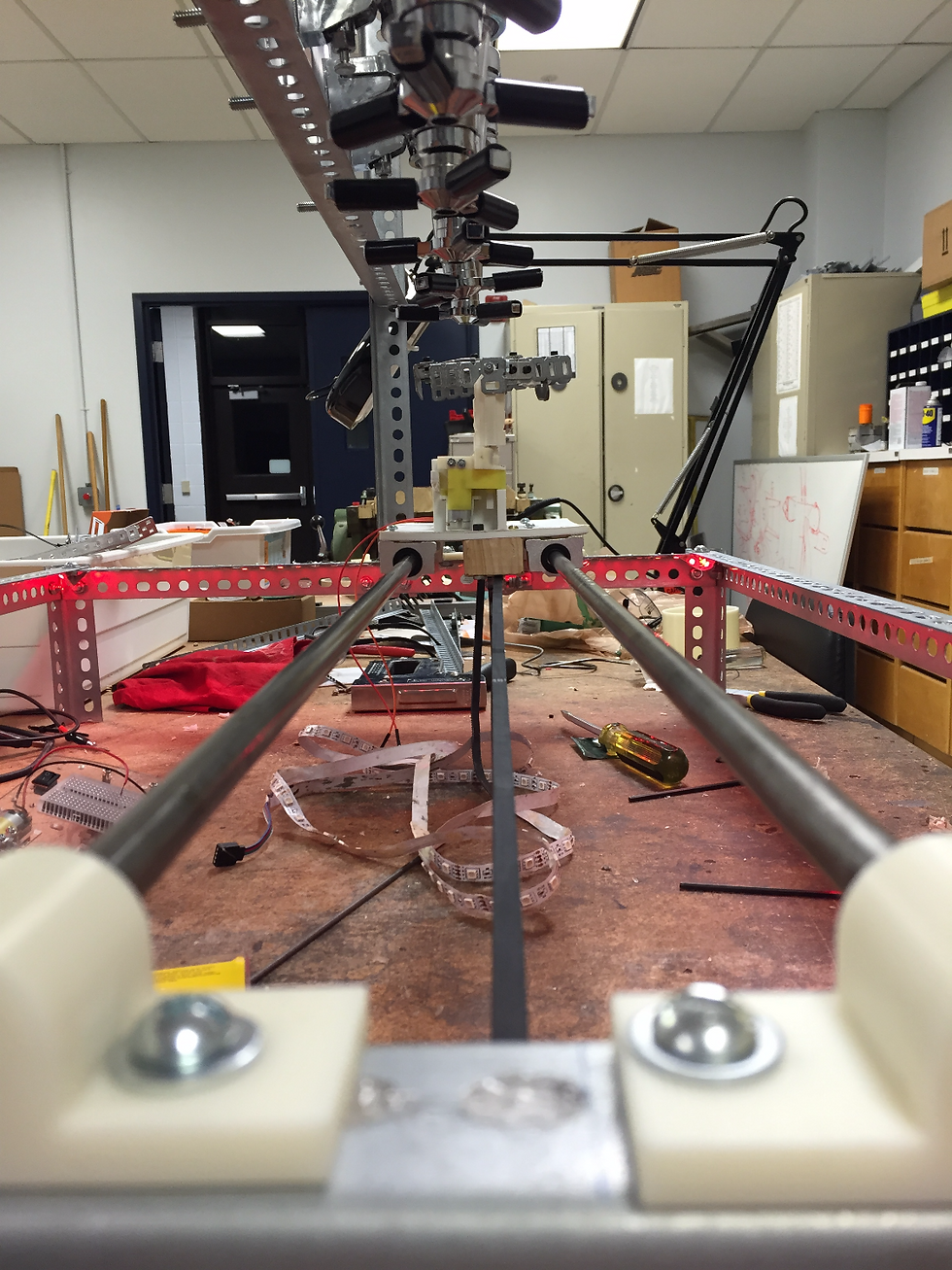
A 4-30 cm SHARP distance sensor is placed on the moving platform to detect if a cup has been placed in the cup platform holder. If no cup is detected, the platform will not pour the drink and will return to it's home position to restart.

The liquor bottle pourer's require a linear force to release the containing beverage. I designed and 3-D printed an attachment to a 5V DC rotational motor to convert its rotational motion into a linear, actuating, motion. This can be seen attached to the moving platform.
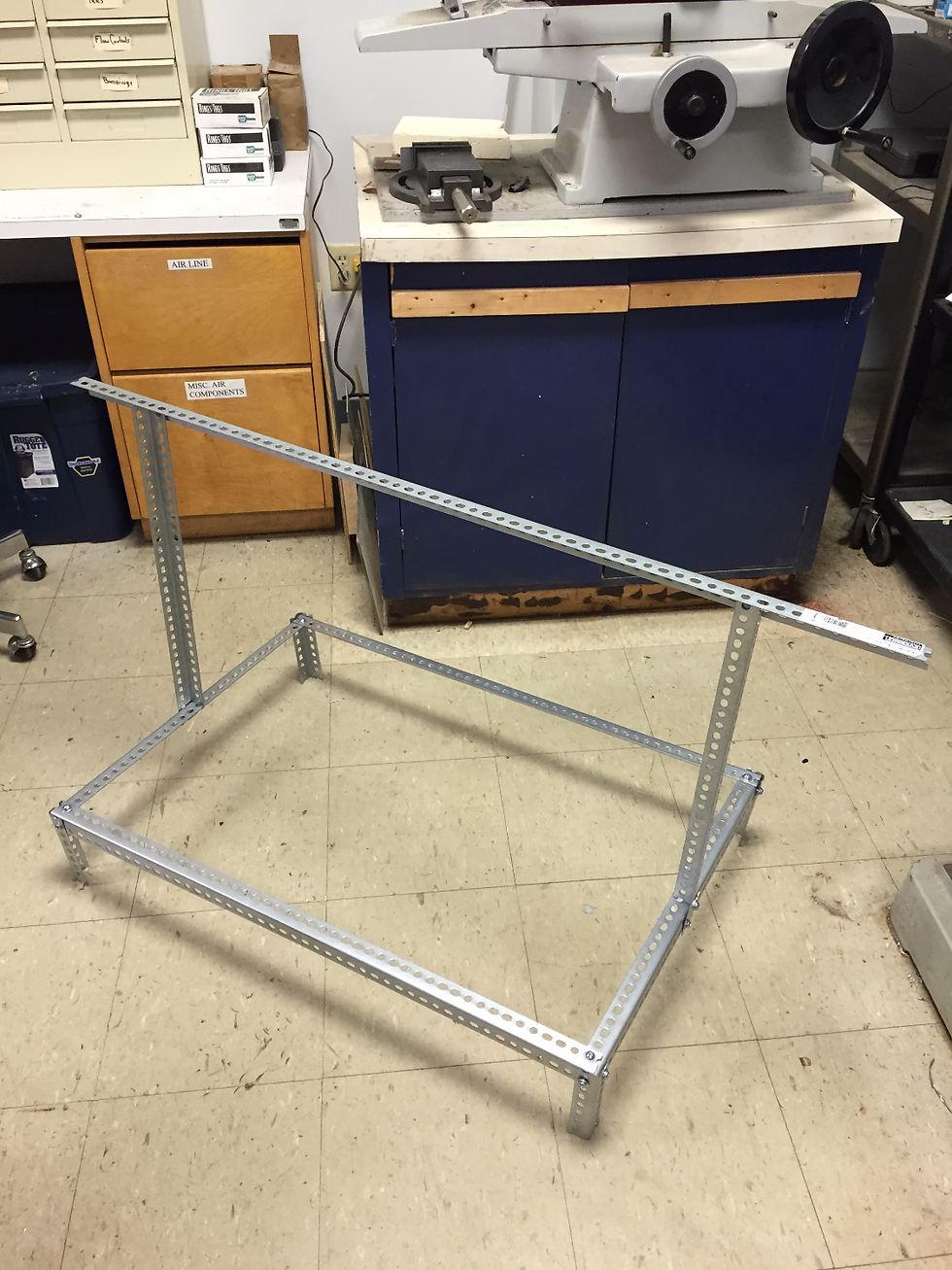
Using a simple aluminium chassis gave the Shot-Bot a strong body while also remaining surprisingly lite. Limited material manipulation required us to use a simple, and unnecessarily large, frame.
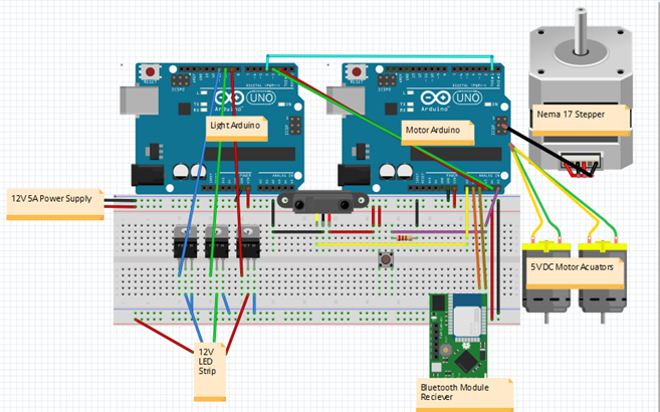
An overview of all the parts can be seen above. A second Arduino was used to solely control the lights and receive commands from the master Arduino, the Motor Arduino.

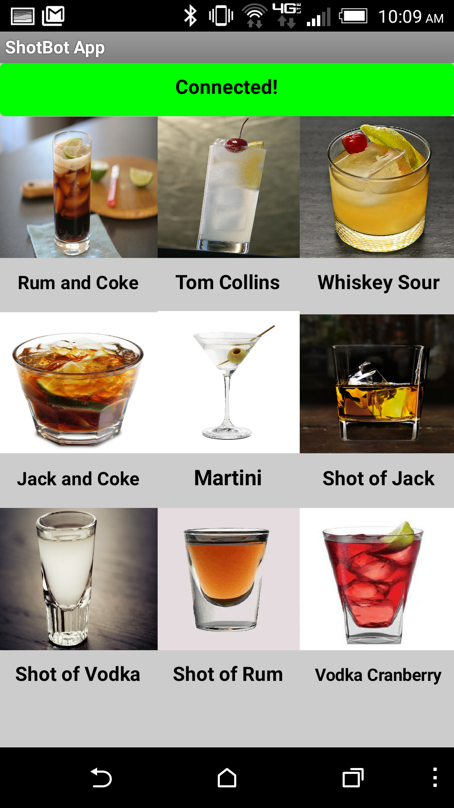
The app is a simple connect and choose style app. It was created using MIT-App inventor 2.0. The receiver module used is the HC-05 Bluetooth module.

The Adafruit motor shield v1 was used to control the stepper and the two DC motors.
Below is the entire code of the system that was written in the Arduino IDE. It is a C based language.
//This code is used to run the Shot-Bot 1.0. // Code is written by Josh Leipheimer
// These are the libraries inlcuded for this software #include <AccelStepper.h> #include <MultiStepper.h> #include <AFMotor.h> #include <SoftwareSerial.h>// import the serial library
SoftwareSerial Genotronex(A1, A2); // A1 goes to Tx and A2 goes to Rx on HC-05 AF_Stepper motor1(200, 2); // (steps per revolution , motor port) AF_DCMotor motorDC(1, MOTOR12_64KHZ); // create DC motor #1, 64KHz pwm AF_DCMotor motorDC2(2, MOTOR12_64KHZ); // create DC motor #1, 64KHz pwm AccelStepper stepper1(forwardstep1, backwardstep1); //Initialized the acceleration and deeceleration for stepper
const int quickstop = 150; //Used to stop DC Motors quicker const int range_up = 4500; //Distance actuator goes up in mS const int range_down = 3800; //Distance actuator goes down in mS
int sensorvalue = 0; //For cup sensor //Drink Positions const int P1=900; //Gin const int P2=1500; //Vodka const int P3=2150; //White Rum const int P4=2800; //Whiskey const int P5=3450; //Coke const int P6=4050; //Sour
int cupsensor = A0; //Distance sesnor pin goes here // These led pins actually send a 5V pulse to the other LED Arduino to tell that Arduino to begin the fading color program const int ledPinCROSSFADE =2; const int ledPinRED = A3; const int ledPinGREEN = A4;
const int buttonPin = A5;
int buttonState = LOW; byte app_number; // The number that the bluetooth module receives to tell the Arduino which drink to make
void setup() { Serial.begin(9600); // set up Serial library at 9600 bps Genotronex.begin(9600); Serial.println("Starting up program"); tiptop1:; // Initialize all pin outputs and inputs and motor speeds pinMode(ledPinCROSSFADE, OUTPUT); pinMode(ledPinRED, OUTPUT); pinMode(ledPinGREEN, OUTPUT); pinMode(buttonPin, INPUT); motor1.release(); motorDC.setSpeed(150); motorDC2.setSpeed(155); stepper1.setMaxSpeed(600.0); //Sets the allowable max speed the stepper will run stepper1.setAcceleration(500.0); // Sets the maximum acceleration and deceleration that the stepper will run buttonState = digitalRead(buttonPin); // check if the pushbutton is pressed. If it is, the buttonState is HIGH:
//Start the Shot-Bot by having a red light to signify it needs calibration digitalWrite(ledPinRED, HIGH); digitalWrite(ledPinGREEN, LOW); digitalWrite(ledPinCROSSFADE, LOW); //User moves the platform to its home position then presses the button to exit calibration mode if (buttonState == HIGH) { // turn Green LED on: digitalWrite(ledPinRED, LOW); digitalWrite(ledPinGREEN, HIGH); goto tiptop3; } else { // Until the button is pressed, the void setup code will continue to loop and prevent the main loop code from starting goto tiptop1; }
tiptop3:; delay(3000); digitalWrite(ledPinGREEN, LOW); //Goes to main loop() code section now }
void loop() { digitalWrite(ledPinCROSSFADE, HIGH); //Start fading LED's motor1.release(); // Releases stepper motor to allow physical movement buttonState = digitalRead(buttonPin); // Waits for user to be ready, once button is pressed again, the actuator will go //up to allow user to place cup if (buttonState == HIGH) { motorDC2.run(RELEASE); // turn it on going forward delay(1000); Serial.println("1"); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(3000); motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); delay(quickstop); Serial.println("2"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); } // Reads blueooth data if (Genotronex.available()) { app_number=Genotronex.read(); switch (app_number) { //lowers actuator motors back to home position, then commences making drink case '1': motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); // turn it on going forward delay(2700); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(quickstop); Serial.println("4"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); drink2(); //Rum and Coke break;
case '2': motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); // turn it on going forward delay(2700); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(quickstop); Serial.println("4"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); drink1(); // Tom Collins break;
case '3': motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); // turn it on going forward delay(2700); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(quickstop); Serial.println("4"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); drink3(); //Whiskey Sour break;
case '4': motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); // turn it on going forward delay(2700); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(quickstop); Serial.println("4"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); drink4(); // Jake and Coke break;
case '5': motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); // turn it on going forward delay(2700); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(quickstop); Serial.println("4"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); drink5(); // Jake and Coke break;
default: //If no viable input is used, default will run and just restart the loop break; } delay(10); }
}
/////////////////Drink 1 ////////////////////
void drink1() //Tom Collins {
int n1=1; sensorvalue = analogRead(cupsensor); while (sensorvalue > 300 && n1==1) // check to see if the cup is on the drink holder { Serial.println("Starting to make Tom Collins");
stepper1.runToNewPosition(P1); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); //check sensor again if(sensorvalue < 300) //if the sensor detects there is no cup, the program will return home { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P1 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top; } Serial.println("Pouring Gin, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P6); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); if(sensorvalue < 300) { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P6 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top; } Serial.println("Pouring Gin, please wait"); DCMotor(); delay(100); stepper1.runToNewPosition(P6 - stepper1.currentPosition()); Serial.println("Finished making Tom Collins"); Serial.println("Final Position:"); Serial.println(stepper1.currentPosition()); // return back to home n1=0; } top:;
}
/////////////////Drink 2 ////////////////////
void drink2() //Rum and Coke {
int n1=1; sensorvalue = analogRead(cupsensor); while (sensorvalue > 300 && n1==1) // check to see if the cup is on the drink holder { Serial.println("Starting to make Whiskey Sour"); stepper1.runToNewPosition(P3); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); //check sensor again if(sensorvalue < 300) //if the sensor detects there is no cup, the program will return home { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P3 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Whiskey, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P5); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); if(sensorvalue < 300) { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P5 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Sour, please wait"); DCMotor(); delay(100); stepper1.runToNewPosition(P5 - stepper1.currentPosition()); Serial.println("Finished making Tom Collins"); Serial.println("Final Position:"); Serial.println(stepper1.currentPosition()); // return back to home n1=0; } top2:;
}
/////////////////Drink 3 ////////////////////
void drink3() //Whiskey Sour { int n1=1; sensorvalue = analogRead(cupsensor); while (sensorvalue > 300 && n1==1) // check to see if the cup is on the drink holder { Serial.println("Starting to make Rum and Coke");
stepper1.runToNewPosition(P4); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); //check sensor again if(sensorvalue < 300) //if the sensor detects there is no cup, the program will return home { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P4 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Rum, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P6); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); if(sensorvalue < 300) { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P6 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Coke, please wait"); DCMotor(); delay(100); stepper1.runToNewPosition(P6 - stepper1.currentPosition()); Serial.println("Finished making Tom Collins"); Serial.println("Final Position:"); Serial.println(stepper1.currentPosition()); // return back to home n1=0; } top2:; }
/////////////////Drink 4 ////////////////////
void drink4() //Whiskey Coke {
int n1=1; sensorvalue = analogRead(cupsensor); while (sensorvalue > 300 && n1==1) // check to see if the cup is on the drink holder { Serial.println("Starting to make Jake and Coke");
stepper1.runToNewPosition(P4); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); //check sensor again if(sensorvalue < 300) //if the sensor detects there is no cup, the program will return home { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P4 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Jake, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P5); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); if(sensorvalue < 300) { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P5 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Coke, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P5 - stepper1.currentPosition()); Serial.println("Finished making Tom Collins"); Serial.println("Final Position:"); Serial.println(stepper1.currentPosition()); // return back to home n1=0; } top2:;
}
void drink5() //Martini { int n1=1; sensorvalue = analogRead(cupsensor); while (sensorvalue > 300 && n1==1) // check to see if the cup is on the drink holder { Serial.println("Starting to make Jake and Coke");
stepper1.runToNewPosition(P1); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); //check sensor again if(sensorvalue < 300) //if the sensor detects there is no cup, the program will return home { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P1 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Jake, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P2); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); if(sensorvalue < 300) { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P2 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Coke, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P6); Serial.println("Current Position: "); Serial.println(stepper1.currentPosition()); sensorvalue = analogRead(cupsensor); if(sensorvalue < 300) { Serial.println("Error: Cup is missing. Returning to Home"); stepper1.runToNewPosition(P6 - stepper1.currentPosition()); Serial.println(stepper1.currentPosition()); goto top2; }
Serial.println("Pouring Coke, please wait"); DCMotor(); delay(100);
stepper1.runToNewPosition(P6 - stepper1.currentPosition()); Serial.println("Finished making Tom Collins"); Serial.println("Final Position:"); Serial.println(stepper1.currentPosition()); // return back to home n1=0; } top2:;
}
void DCMotor() //This is the function that runs the actuator to pour the drinks { motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(1000); Serial.println("1"); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(range_up); motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); delay(quickstop); Serial.println("2"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(4000); Serial.println("3"); motorDC.run(BACKWARD); // turn it on going forward motorDC2.run(BACKWARD); // turn it on going forward delay(range_down); motorDC.run(FORWARD); // turn it on going forward motorDC2.run(FORWARD); // turn it on going forward delay(quickstop); Serial.println("4"); motorDC.run(RELEASE); // turn it on going forward motorDC2.run(RELEASE); // turn it on going forward delay(2000); Serial.println("5"); }
void forwardstep1() //This is used to give the stepper its acceleration and decceleration abilities { motor1.onestep(FORWARD, DOUBLE); } void backwardstep1() { motor1.onestep(BACKWARD, DOUBLE); }
Comments